Living without zabbix-sender
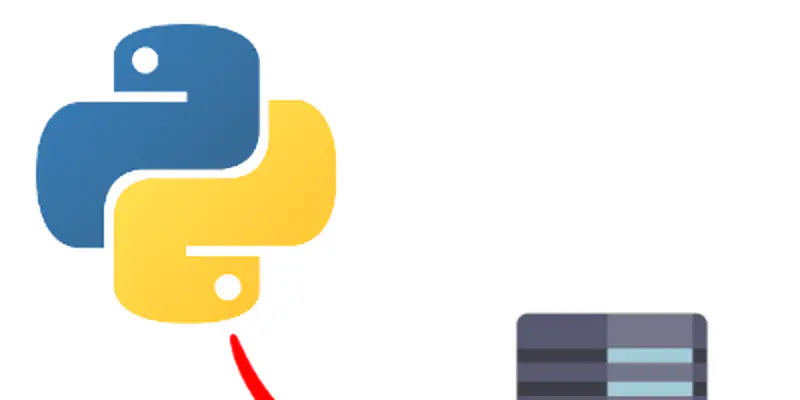
If you like Zabbix and you’re using it, you know that sometimes you need to send a value from the monitored host to the zabbix server/proxy. One of the most common scenario, for me, is the status of a backup job when this is managed by the host: at the end of the job I usually call a script that sends the backup status (OK or KO) through zabbix-sender. Like this:
1do_backup && STATUS=OK || STATUS=KO
2zabbix_sender --zabbix-server $ZABBIX_SERVER --host "$ZABBIX_HOSTNAME" \
3 --key $ZABBIX_HOSTKEY --value "Backup $STATUS"
On the zabbix server side, there’s an item of type “trapper” in the corresponding host, which will be updated. And with a couple of triggers (“backup error” and “no good backups in the last 12 hours”) we can sleep well.
You don’t need the sender
Sadly, sometimes, you can’t have the zabbix_sender. Typically because you’re on a strange architecture, or have very limited resources, or running on an AS/400.
Here’s a workaround script, in python, that doesn’t need to be fed into the zabbix_sender. This is based on this protocol.
1#!/usr/bin/env python
2
3import datetime
4import json
5import socket
6
7ZABBIX_SERVER = '333.12.33.44'
8ZABBIX_HOSTNAME = 'TEST'
9ZABBIX_ITEMKEY = 'test'
10PORT = 10051
11BUFFER_SIZE = 1024
12
13# just replace this with the desired value
14msg = datetime.datetime.now().strftime("Message timestamp: %Y/%m/%d-%H:%M:%S")
15
16msg_json = json.dumps({
17 "request":"sender data",
18 "data":[
19 {
20 "host": ZABBIX_HOSTNAME,
21 "key": ZABBIX_ITEMKEY,
22 "value": msg
23 }
24 ]
25})
26
27sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
28
29(family, socktype, proto, garbage, address)=socket.getaddrinfo(HOST, PORT)[0]
30sock = socket.socket(family, socktype, proto)
31sock.connect(address)
32sock.send(msg_json)
33recv_data = sock.recv(BUFFER_SIZE)
34sock.close()
35
36# you don't really need to print
37# you're receiving something like this:
38# ZBXDZ{"response":"success","info":"processed: 1; failed: 0; total: 1; seconds spent: 0.000200"}
39print "received data:", recv_data